XML Document Object Model
In This Topic
The XML Document Object Model is an in-memory tree representation of an XML document and allows for easy navigation and manipulation of this document. The XML DOM provided by Nevron Open Vision is built around the NXmlDocument, NXmlNode and NXmlElement classes. The following diagram illustrates the class hierarchy of the Nevron XML DOM:
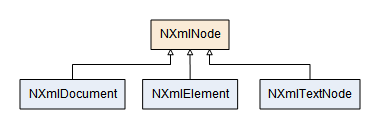
The sections below describe the functionality these classes provide in more details.
Xml Nodes
NXmlNode is the base abstract class, which all XML DOM objects inherit. Any XML node has a name, a type, a reference to its parent XML node, a list of children and a list of declarations. Nevron Open Vision supports the following types of XML nodes:
XML Node Type |
Description |
Document |
Represents a XML document. |
Element |
Represents a XML element. XML elements are XML nodes, which can have attributes. |
Declaration |
Represents a XML declaration, for example - <?xml version="1.0" encoding="UTF-8"?> |
Text |
Represents a XML text node, which contains the text content of a XML element. |
Comment |
Represents a XML comment, i.e. a XML text node surrounded by "<!--" and "-->". |
CDATA |
Represents a XML data section, i.e. a XML text node surrounded by "<![CDATA[" and "]]>". |
The list below describes how to perform the most common actions on XML nodes:
- Navigate to the parent node - use the Parent property of the node to get the node the current node is currently placed in. If the value of the Parent property is null, then the current node is the root node.
- Navigate to an ancestor node - use one of the GetFirstAncestor methods to navigate to the first ancestor with a given name.
- Navigate to a child node - use the GetChildAt method to navigate to the child of the node at a given index or the GetChildByName method to navigate to the child with a given name.
- Navigate to a descendant node - use the GetFirstDescendant method to get the first descendant with a given name.
- Get all descendants with a given name - use the GetDescendants method to get a list of all descendants with a given name.
- Add/Remove a child node - use the AddChild or InsertChild methods to add a child to the current node and the RemoveChild method to remove a child node from it.
- Add/Remove a declaration - use the AddDeclaration and RemoveDeclaration methods
Xml Text Nodes
XML text nodes are XML nodes that contain text data. They are usually leaf nodes in the DOM tree (i.e. they do not have any child nodes) and the only properties of interest for them are the Text property, which contains the text data of the node and the NodeType property, which indicates the type of the node. XML text nodes can be of type Text, Comment and CDATA.
Xml Elements
XML elements are XML nodes, which can have attributes. To create a XML element use any of the constructors of the NXmlElement class:
- NXmlElement(string name) - creates a XML element with the given name.
- NXmlElement(string name, string value) - creates a XML element with the given name and adds a text node child with the given content.
XML elements usually contain other elements or text nodes. To get the concatenated values of all child nodes of an XML element, use its InnerText property. You can also use the InnerText property of the XML element to set its text. This will remove all child nodes of the XML element and replace them with a single text node that contains the specified text.
To determine how many attributes an XML element has, use the AttributeCount property. Through the SetAttribute method you can add/update an attribute name/value pair to the XML element. The RemoveAttribute method removes an attribute from the element and the ClearAttributes method removes all attributes from it. If you want to get the value of an attribute with a given name, simply call the GetAttribute method.
The GetAttribute method returns null if the XML element does not contain an attribute with the given name.
To loop through the attributes of an XML element, you should use the GetAttributesIterator method to obtain an attributes iterator:
Iteration over XML element's attributes |
Copy Code
|
INIterator<NKeyValuePair<string, string>> iter = xmlElement.GetAttributesIterator();
while (iter.MoveNext())
{
string attributeName = iter.Current.Key;
string attributeValue = iter.Current.Value;
// Process the attribute here
}
|
Xml Document
The NXmlDocument is a XML node that is usually the root node of every XML DOM tree. You can navigate and manipulate its children in the same way you do for XML nodes. The NXmlDocument class extends the NXmlNode class with the ability to load and save XML documents from and to a stream:
- Loading of XML documents - to load a XML document from a stream, use the LoadFromStream static method of the NXmlDocument class. This method returns a XML document result of the parsing of the given stream of XML data, or null if the given stream does not contain valid XML data. Alternatively, you can use the LoadFromFile and LoadFromString static methods of the NXmlDocument class.
- Saving of XML documents - to save a XML document to a stream, use the SaveToStream method of the XML document instance. You can optionally specify an encoding for the XML data. If you don't specify an encoding UTF-8 will be used. Alternatively you can use the SaveToFile and SaveToString methods.
The following piece of code demonstrates how to create a XML document and save it to a file:
Creating and saving a XML document |
Copy Code
|
// Create a simple XML document
NXmlDocument xmlDocument = new NXmlDocument();
NXmlElement bookElement = new NXmlElement("Book");
bookElement.SetAttribute("PublishDate", "1990");
xmlDocument.AddChild(bookElement);
// Create an XML element that contains some text
NXmlElement titleElement = new NXmlElement("Title");
titleElement.AddChild(new NXmlTextNode("Jurassic Park"));
bookElement.AddChild(titleElement);
// Shortcut method for creating an XML element with text
NXmlElement authorElement = new NXmlElement("Author", "Michael Crichton");
bookElement.AddChild(authorElement);
// Save the XML document to file
xmlDocument.SaveToFile(NFileSystem.GetFile(@"D:\Book.xml"));
|
This code will produce the following XML:
Resulting XML |
Copy Code
|
<?xml version="1.0" encoding="UTF-8"?>
<Book PublishDate="1990">
<Title>Jurassic Park</Title>
<Author>Michael Crichton</Author>
</Book>
|
See Also